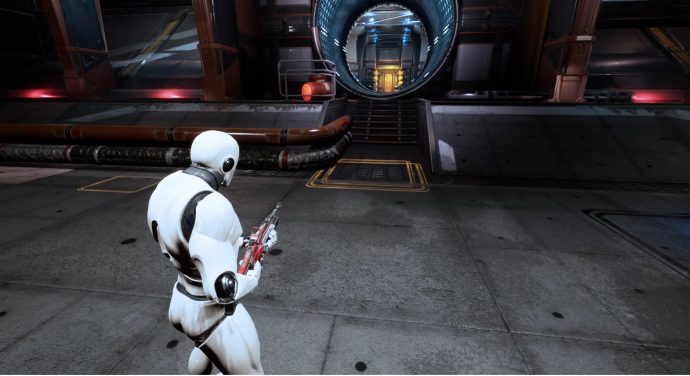
Camera for an Horror Game in UE4
After more than 5 years without any post or updates in this blog, I decided to write again. I have two games in development, one for mobile platforms in Unity, and one game for PC in UE4. I’m also composing music with Sonic-PI and experimenting with Algorave, and I decided to write something about these projects. I will write about the mobile game and Sonic-PI experiments in another future posts. In this one, I will write about some tests I’m doing with horror gameplay.
There are many things you have to do write when you make a game. For a horror game, I think the first one to give the game experience you want is to have the correct camera.
We are aiming to a type of camera like the Dead Space series, where the player can rotate the camera around the character when she is not moving the pawn and, as soon the character starts moving, it aligns with the camera forward direction. When the player is aiming, the character can also move backwards without having to change direction.
This is really easy to achieve with the Third Person template of the Unreal 4 engine. The key property is the UseControlledDesiredRotation variable of the CharacterMovement component. If you leave this variable unchecked, you can rotate the camera around the character without having it to align with the camera forward direction. Then, you can write some code to set this variable to true when the character starts moving.
In the Dead Space series, there’s also the aiming interpolation of the camera from the default position to somewhere closer to the shoulder of the character. It’s also easy to reproduce this with Timeline in Blueprint or with the FMath::FInterpTo function in C++.
C++ Code
The complete code you can find in this github link. (The screenshots with the Blueprint version is also available in this link) The most important part of the code is the AlignPawnToCameraDir() function and the use of the interpolation in the Tick() function.
The Tick() function is shown bellow
void APlayerCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
AlignPawnToCameraDir();
if (!FMath::IsNearlyEqual(CameraBoom->TargetArmLength, DesiredSpringArmLength, 0.5F))
{
CameraBoom->TargetArmLength = FMath::FInterpTo(
CameraBoom->TargetArmLength,
DesiredSpringArmLength,
DeltaTime,
ZoomSmoothness);
}
}
For the aiming part, I created a new variable, DesiredSpringArmLength, that is set to the default camera position when the player is not aiming, and set to the over the shoulder position when the player is aiming. There’s an Input Event to set this value that is called when the player is aiming. Then, I check if the actual ArmLength of the CameraBoom component needs to be updated and interpolate between this position and the correct one.
The AlignPawnToCameraDir() function just check if the Forward or Backward values have changed through Input events, if they are not zeros, or if the the player is aiming, and set the UseControlledDesiredRotation property of the CharacterMovement to the correct value.
void APlayerCharacter::AlignPawnToCameraDir()
{
bool use = ForwardValue != 0.0F || RightValue != 0.0F || bIsAiming;
GetCharacterMovement()->bUseControllerDesiredRotation = use;
}
Blueprint
The blue print blocks are displayed bellow. I show only the portions of the code that matters to this camera topic. The others are the default ThirdPersonBP template.
There are two main differences from C++: I check if the character needs to realign itself with the camera forward direction after each Input and not in the Tick() event, and I used a Timeline to make the interpolation from the camera position to the desired one when the player is aiming the gun. These screenshots are also available in the github link for the C++ code.
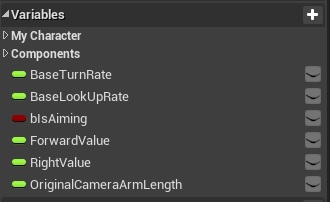
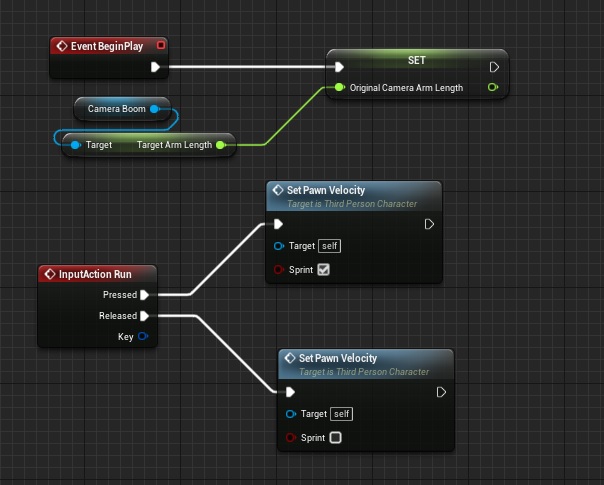
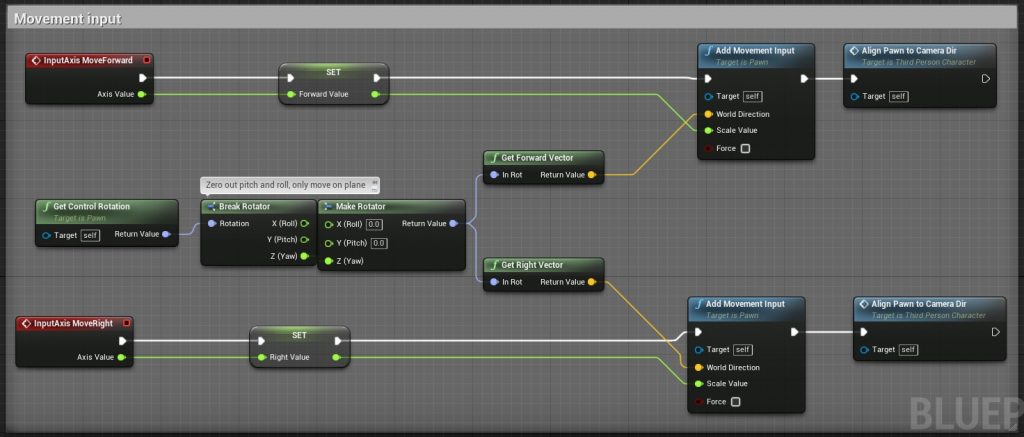
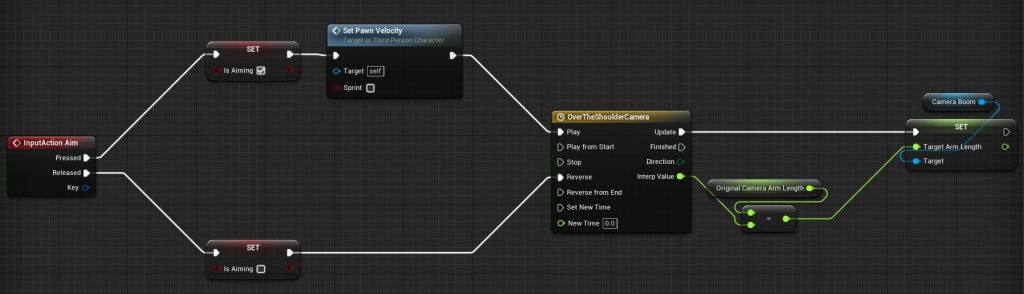
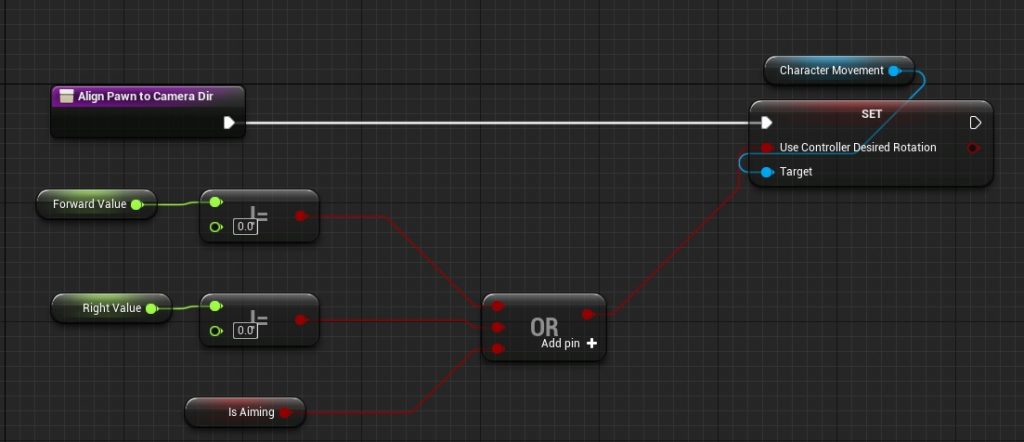
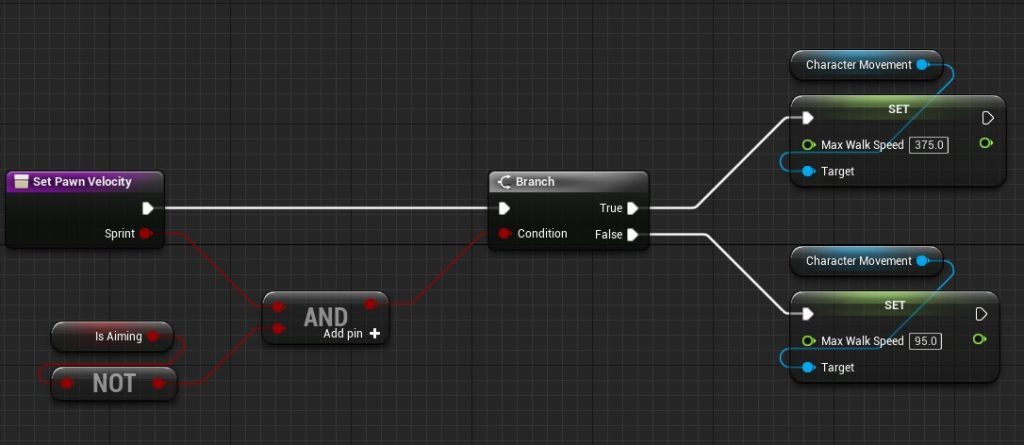
The video bellow is a test I did with the code above. The environment is the Scifi Kitbash by Denis Rutkovsky. Epic made this asset free a few months back, and I used it to test the camera code.
If you have any question, just use the contact form and send me an email. Thanks for reading until the end.
End of Line